This article explains how to automatically adjust the font size relative to the widgets in Kivy.
Responsive Text in Kivy Apps
Kivy application automatically make widgets such as labels and buttons responsive, but they do not automatically adjust the size of text. As shown in the figure below, the text protrudes from the button when the screen size is changed.
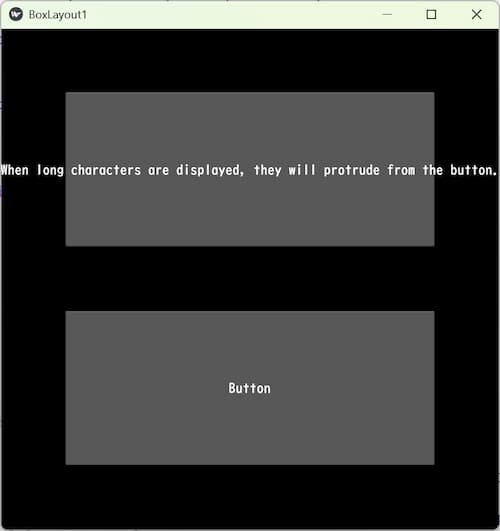
The example shown here resizes the text relative to the size of the widget. It also wraps the text so that the string fits within the widget. If you do not do this, the text will extend beyond the widget. Try resizing the screen to test it.
text_responsive.py
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
class RootWidget(BoxLayout):
pass
class TextResponsive(App):
def build(self):
return RootWidget()
if __name__ == "__main__":
TextResponsive().run()
textresponsive.kv
<RootWidget>:
orientation: "vertical"
padding: sp(10)
spacing: sp(10)
Label:
text: "The text will automatically adjust to the widget size!"
#size_hint_y: None # Fixed size.
#height: self.texture_size[1] # Match height to text content.
text_size: self.width, None # Specify width to wrap text.
halign: "center" # Horizontal text position.
valign: "middle" # Vertical position of text.
font_size: min(sp(25), self.width * 0.07) # 25sp minimum or 7% of widget width.1
Button:
text: "The text will automatically adjust to the size of the widget!"
size_hint: 1, None
#height: sp(100) # Fix button height.
#text_size: self.size # Fit text to button size.
text_size: self.width, None # Specify width to wrap text.
halign: "center" # Horizontal text position.
valign: "middle" # Vertical position of text.
#font_size: min(sp(20), min(self.width, self.height) * 0.05) # Adjustment with width and height and minimum value 15dp.
font_size: min(sp(25), self.width * 0.07) # 25sp minimum or 7% of widget width.
Code Explanation
This example is set up to automatically adjust the font size of text relative to the widget. When the screen size changes, the text size is adjusted, and if the text does not fit in the widget, the text is wrapped.
sp(100)
This writing is to convert the units and should be changed if you want to use dp, etc. I think the default unit for font size is sp. The units of padding and spacing used for layout and such are pixels.
size_hint_y: None
height: self.texture_size[1]
The size_hint_y sets how much height is allocated to the widget relative to the parent widget’s space; setting it to None will not allocate a minimum height. If a height is set together with the size_hint_y, that value will be used. The usage of size_hint can be found in the following article.
size_hint: 1, None
height: sp(100)
The size_hint sets how much space to allocate to the widget relative to the parent widget’s space. Here the height is set so the height is fixed at 100 and the horizontal size is 100%.
text_size: self.width, None
text_size: self.size
The text_size sets the text drawing range in [width, height].
- If [None, None], the text will be sized to fit within the drawing area; otherwise, the text will be cropped to fit within the drawing area.
- Wrap the text to fit the width of the widget by setting [self.width, None]. self.width gets the width of its own widget.
- By setting [self.size], the text is adjusted to fit the size of the widget. self.size retrieves the size of its own widget.
halign: "center" # horizontal position of text.
valign: "middle" # vertical position of text.
Sets the vertical and horizontal position of the text.
- The halign specifies the horizontal position of the text as [auto, left, center, right, justify]. justify aligns the lines if there are multiple lines. The default is auto.
- The valign specifies the vertical position of the text as [bottom,middle (or center ),top]. The default is bottom.
font_size: min(sp(20), min(self.width, self.height) * 0.05) # minimum width and height or 20dp.
font_size: min(sp(25), self.width * 0.07) # min 25sp or 7% of widget width.
Sets the font size. min sets the minimum value, and the smaller of the two arguments is used.
font_size: min(sp(20), min(self.width, self.height) * 0.05) # minimum width and height or 20dp.
- Set the minimum value to 20; if smaller, use 5% of the Button’s width or height.
- min(self.width, self.height) is the smaller of width and height.
font_size: min(sp(25), self.width * 0.07) # min 25sp or 7% of widget width
- Set the minimum value to 25; if it is smaller, use 5% of the Label width.
Please also refer to the following article for more information on text-related properties that can be set. (This article has not been translated yet.)
Comment