This section explains how to use pos or pos_hint to set the widget position in the Kivy application. These are limited to use with some layout widgets. It also explains how to get the position of a parent or children.
Setting the Widget Position
Use pos or pos_hint to set the widget position. These properties can be used with some layout widgets.
- BoxLayout (may not work correctly)
- GridLayout
- Float Layout
- RelativeLayout
- ScatterLayout
Set the pos or pos_hint property on the widget.
The pos_hint Property
The x-axis (horizontal) and y-axis (vertical) specify the position of the widget; in the case of Kivy, the lower left corner of the screen is x=0 and y=0. The value is specified as a number between 0 and 1, with 0.5 being 50%.
- Specify by x-axis and y-axis
- center_x andcenter_y
- position_right andposition_top
The pos_hint on the x- and y-axis
Specify the widget’s position on the x-axis (horizontal) and y-axis (vertical); in the case of Kivy, the lower left corner of the screen will be x=0, y=0, and a number between 0 and 1. 0.5 is 50%.
If padding, which sets the inner margin of the layout, or spacing, which sets the space between widgets, is set, these values are excluded from the calculation.
For example, if x=0.3 and y=0.6, the widget will be placed 30% from the left on the x-axis and 60% from the bottom on the y-axis.
pos_hint {'x': 0.5, 'y': 0.5}
If x=0.5 and y=0.5 as shown above, the widget is not placed in the center of the screen. This is because the widget is positioned based on a different base position for each of the three methods, and when specified on the x and y axes, the widget is positioned with respect to the lower left corner of the widget. In other words, the lower left corner of the widget is positioned 50% from the left and 50% from the bottom. Thus, the widget will appear off-center rather than centered on the screen. To center the widget, use center_x and center_y.
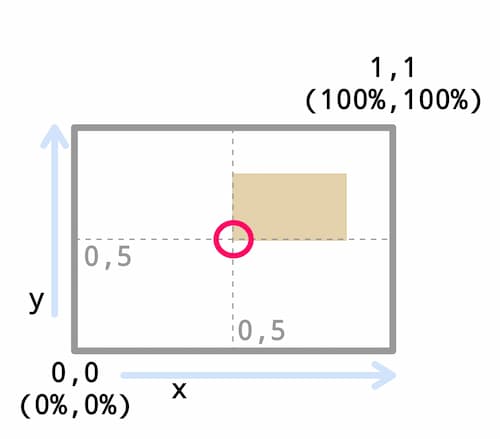
Specifying pos_hint With center_x and center_y
The center_x and center_y are also specified in the same way, using a number between 0 and 1. The base position of this is the center point of the widget. For example, x=0.1 and y=0.3 will place the center point of the widget at 10% from the left on the x-axis and at 30% from the bottom on the y-axis. x=0.5 and y=0.5 will place the widget at the center of the screen.
pos_hint: {'center_x': 0.5, 'center_y': 0.5}
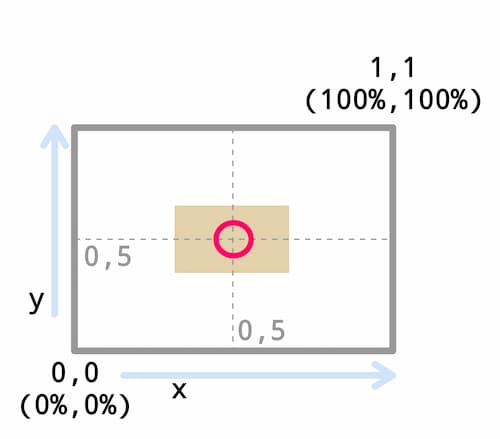
Specifying pos_hint With right and top
You can also specify pos_hint by right and top. The base position for this is the upper right corner of the widget.
pos_hint: {'right': 0.5, 'top': 0.5}
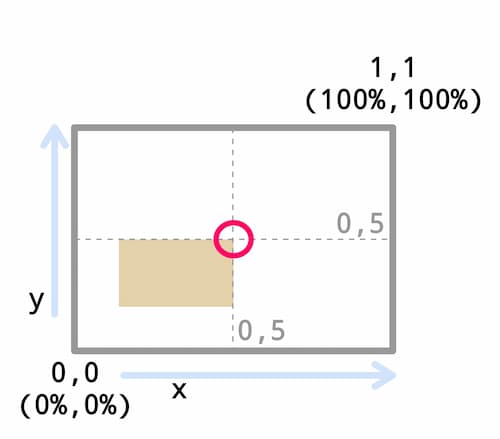
Summary of Widget Base Position
Keys Can Be Mixed
It is also possible to write a mixture of [x , y] and [right , top] keys.
# Placement on top left corner.
pos_hint={'x': 0, 'top': 1}
# Placement in bottom right corner.
pos_hint={'right': 1, 'y': 0}
# Specified by mixing center_x and y.
pos_hint: {'center_x': 0.5}
y: 100
The pos Property
The pos property specifies the x- and y-axis coordinates.
pos: 300, 500
Widgets That Extend Beyond the Screen
If a widget is placed beyond the boundaries of the screen size, it will be displayed out of the widget screen. Note that no error will be thrown.
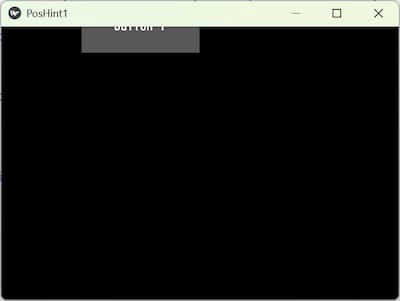
Code Example
pos_hint1.py
from kivy.app import App
from kivy.uix.relativelayout import RelativeLayout
class RootWidget(RelativeLayout):
pass
class PosHint1(App):
def build(self):
return RootWidget()
if __name__ == '__main__':
PosHint1().run()
poshint1.kv
<RootWidget>:
padding: 20
#spacing: 20
Button:
text: 'Button 1'
size_hint: 0.3, 0.2
#pos_hint: {'x': 0.2, 'y': 0.9} # This will cause the widget to protrude from the screen.
pos_hint: {'center_x': 0.5, 'center_y': 0.5}
#pos_hint: {'right': 0.5, 'top': 0.5}
#pos_hint: {'x': 0, 'top': 1}
#pos: 300, 500
#pos_hint: {'center_x': 0.5}
#y: 100
Get Widget Position
Here are a few ways to get the position of a widget. This is useful when you want to calculate the position of a widget based on the position of another widget.
Getting the [ x , y ] position of the widget returns the origin (lower left corner). The [ x , y ] of the parent will be returned [ 0 , 0 ] and the [ x , y ] of the child will be the position of the lower left corner of the widget.
If the widget’s [ center-x , center-x ] position is retrieved, the position of the widget’s center is returned.
Get the Position of Its Own Widget With KV Code
The self keyword is used to get the widget of itself in kv code. Usually, it is passed as an argument or used to display its own size in text.
Button:
pos_hint: 1, 0
text: str(self.pos)
on_press: callback_function(self.pos)
Get the Position of Other Widgets With KV Code
Use id to get the position of other widgets in kv code.
Button:
id: button
text: 'text'
pos_hint: {'center_x': 0.5, 'y': 0.9}
size: 100,50
Label:
text: 'text'
pos_hint: {'center_x': 0.5}
y: int(button.y - 140)
In the above example, the vertical position is obtained from the Button id. 0.5 (center) is specified for the x-axis, the y-axis is based on the Button position, and 140 is subtracted from that value. button.y is returned as a flot type, so it is converted to an int type.
Get the Position of Parent With KV Code
To get the position of the parent in the kv code, use the parent keyword.
Button:
on_press:
print(f"Parent pos-x: {self.parent.x} pos-y: {self.parent.y}")
print(f"Parent.pos: {self.parent.pos}")
When this is retrieved, [0, 0], the origin of the parent (lower left corner), is returned.
Get the Position of Children With Python Code
Since the root widget is the parent, the widgets configured in its tree are children. Child widgets can be retrieved at once in a list of children.
for child in self.children:
print(f"Child {child.text} size: {child.size}, pos: {child.pos}")
The children are listed below and are expanded with a for statement. You can retrieve the widget’s properties using the temporary variable to which you assigned the object.
[<kivy.uix.label.Label object at 0x000002A2F9CB9400>, <kivy.uix.label.Label object at 0x000002A2EED82C80>, <kivy.uix.label.Label object at 0x000002A2EED80590>, <kivy.uix.label.Label object at 0x000002A2EED6DE10>, <kivy.uix.label.Label object at 0x000002A2EED63690>, <kivy.uix.label.Label object at 0x000002A2EED61080>, <kivy.uix.button.Button object at 0x000002A2EED3E040>]
If you want to get the position of individual child widgets, get them in the ids namespace.
print(f"Child size: {self.ids.button.size}, pos: {self.ids.button.pos}")
Get the Position of Parent With Python Code
Use the self keyword since the root widget is the parent and refers to itself.
print(f"Parent size: {self.size}, pos: {self.pos}")
When this is retrieved, [0, 0], the origin of the parent (lower left corner), is returned.
The following article shows an example of code to get the position and size of other widgets.
Comment