This section describes how to use Kivy’s AnchorLayout, which positions widgets in a fixed center, top, bottom, left, or right position.
What Is AnchorLayout?
AnchorLayout is a layout widget that places widgets in fixed positions (top, bottom, left, right, or center); when AnchorLayout is used by itself, only one widget can be placed. If you want to place multiple widgets, you may want to use it with other layouts or choose other layouts.
Placing Widgets in AnchorLayout
Use the anchor_x and anchor_y properties to set the widget’s position, where x is the horizontal axis and y is the vertical axis.
- anchor_x: [ ‘left‘, ‘center‘, ‘right‘ ] sets the horizontal position. Default is center.
- anchor_y: [ ‘top ‘, ‘center ‘, ‘bottom ‘ ] sets the vertical position. Default is center.
The figure below shows the position of anchor_x and anchor_y.
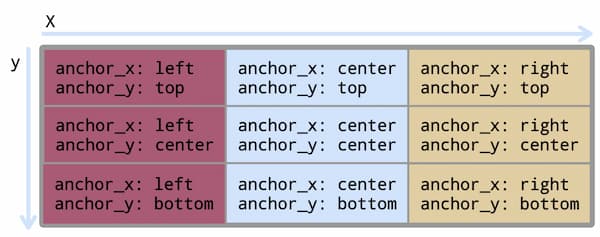
Let’s take a look at the AnchorLayout a code of example.
anchorlayout1.py
from kivy.app import App
from kivy.uix.anchorlayout import AnchorLayout
class RootWidget(AnchorLayout):
pass
class Anchorlayout1(App):
def build(self):
return RootWidget()
if __name__ == '__main__':
Anchorlayout1().run()
anchorlayout1.kv
<RootWidget>:
anchor_x: 'center'
anchor_y: 'top'
padding: 100 # Set the inside margins.
# 背景色の設定
canvas.before:
Color:
rgba: 229/255, 234/255, 230/255, 1
Rectangle:
size: self.size
pos: self.pos
Button: # This is hidden because it overlaps with Button2.
text: 'Button 1'
size_hint: 0.3, 0.2
Button:
text: 'Button 2'
size_hint: 0.3, 0.2
In the above code, Button1 is hidden because Button1 and Button2 are displayed on top of each other. The pos and pos_hint widget positioning properties are not available, so if you want to use multiple widgets, you must nest other layouts. This is explained later in this article.
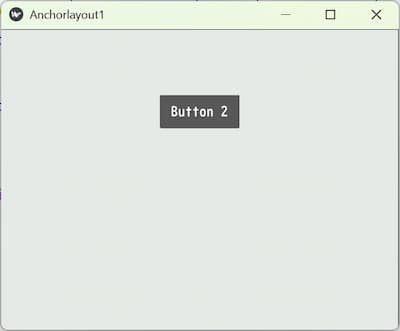
AnchorLayout Settings
AnchorLayout allows you to set the inner margin (padding ) and background color.
Setting the Inner Margin (padding)
To set the inner margin of an AnchorLayout, use the padding property.
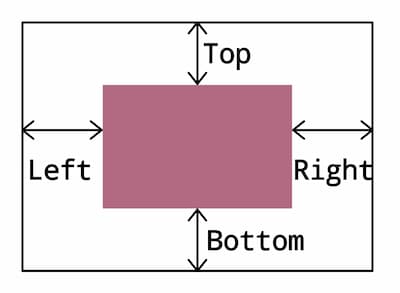
For more information on padding, see the BoxLayout article.
Setting the Background Color
AnchoLayout does not have a property to set the background color, so use canvas. See the following article for details on how to set the background color.
How to Place Multiple Widgets in AnchorLayout
To place multiple widgets in an AnchorLayout, nest other layouts.
The following is an example of code that nests a BoxLayout into an AnchorLayout and places multiple widgets.
anchorlayout2.py
from kivy.app import App
from kivy.uix.anchorlayout import AnchorLayout
class RootWidget(AnchorLayout):
pass
class Anchorlayout2(App):
def build(self):
return RootWidget()
if __name__ == '__main__':
Anchorlayout2().run()
anchorlayout2.kv
<RootWidget>:
anchor_x: 'center'
anchor_y: 'center'
padding: 20
BoxLayout:
spacing: 20
Button:
text: 'Button 1'
size_hint: 0.3, 0.2
pos_hint: {'center_x': 0.5, 'center_y': 0.5}
Button:
text: 'Button 2'
size_hint: 0.3, 0.2
pos_hint: {'center_x': 0.5, 'center_y': 0.5}
This example uses pos_hint to center the widget on the screen.
For more information on pos_hint, please see the following article.
Setting the Widget Size
To set the size of a widget, use the size_hint property.
Comment