This section explains how to set the background color of layout and label in Kivy. There is no such property as background-color for layout and Label widgets. However, the Button widgets has a backgroud_color property, so use that.
Setting the Layouts and Labels Background Color
There are currently two ways to set the layout background color. I am not sure which one is better, but the official site explains how to use canvas to apply the color.
- How to fill the layout with color in canvas
- How to set the screen color in the Window class
How to Use canvas to Fill Colors in a Layout
The canvas is a function for drawing using the Graphics class. The method to set the background color is to draw a rectangle on the layout and fill it with a color.
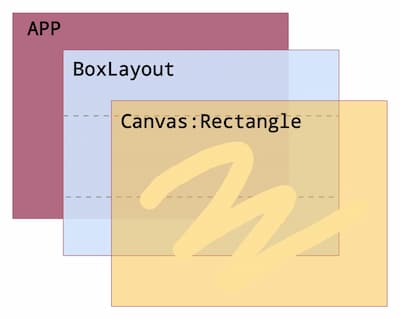
Code Example
This is a sample of painting a background using canvas. For details on specifying colors, please refer to the following page.
background_color1.py
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
class RootWidget(BoxLayout):
pass
class BackgroundColor1(App):
def build(self):
return RootWidget()
if __name__ == "__main__":
BackgroundColor1().run()
background_color1.kv
#:import hex kivy.utils.get_color_from_hex
<RootWidget>:
orientation: 'vertical'
canvas.before:
Color:
rgba: hex("#d8bfd8") # Specify in hexadecimal.
#rgba: 42/255, 100/255, 89/255, 1 # Specify in RGBA.
Rectangle:
size: self.size
pos: self.pos
Label:
text: 'label'
font_size: 50
color: 1, 0, 0, 1
Explanation of Code
#:import hex kivy.utils.get_color_from_hex
rgba: hex("#d8bfd8") # hexadecimal
This is a sample for hexadecimal, so delete it if you want to use RGBA.
canvas.before:
Color: rgba: 42/255
rgba: 42/255, 100/255, 89/255, 1 # RGBA
Rectangle: self.size
size: self.size
pos: self.pos
The canvas.before is a property for creating a drawing block. When this block is created, it is executed first before the widget is drawn. In the case of this code, Rectangle and Color are specified to create a rectangle before the widget is drawn so that it can be used as a background.
The background color is specified in RGBA for Color. Transparency did not work, so hexadecimal might be better for pale color, etc.
The Rectangle.size specifies the size of the Rectangle. Normally, width and height are specified, but by specifying self.size, the size of the widget is obtained and the size of the entire screen area is specified. The widget that corresponds to self here is the RootWidget (=BoxLayout).
The Rectangle.pos specifies the position of the Rectangle. Normally, x and y coordinates are specified, but self.pos also obtains the position of its own widget in the same way.
How to Set the Screen Color in the Window Class
Next, we will show how to set the background color in the Window class.
Code Example
background_color2.py
from kivy.app import App
from kivy.uix.boxlayout import BoxLayout
from kivy.core.window import Window
from kivy.utils import get_color_from_hex
#Window.clearcolor = (42/255, 100/255, 89/255, 1) # Specify in RGBA
Window.clearcolor = get_color_from_hex("#d8bfd8") # Specify in hexadecimal
class RootWidget(BoxLayout):
pass
class BackgroundColor2(App):
def build(self):
return RootWidget()
if __name__ == "__main__":
BackgroundColor2().run()
background_color2.kv
<RootWidget>:
orientation: 'vertical'
Label:
text: 'label'
font_size: 50
color: 1, 0, 0, 1
Explanation of Code
from kivy.core.window import Window
Window.clearcolor = (42/255, 100/255, 89/255, 1) # RGBA
The Window class is imported and the screen color is set with Window.clearcolor.
from kivy.utils import get_color_from_hex
Window.clearcolor = get_color_from_hex("#d8bfd8") # hexadecimal
To set the hexadecimal number, use the get_color_from_hex() method of the utils class.
See the following article for other settings of Window class.
Set a Background Image for the Layout
See the following article for more information on how to use images as backgrounds for layouts.
Comment